Introduction
A program is an executable stored in a physical disc. Say for example Notepad.exe stored in the Windows System32 folder is called the Notepad program. When the program in secondary storage (Disc) loads into primary storage for the execution of instructions set in it, we call it a process. Windows has the capability of spawning multiple processes at a time and we call Windows a multi-tasking operating system. When all processes running in the background they compete for the shared resources. OK, this article we will see how to spawn a process from a C# application.
About the Example
The following screen shot shows the application example:
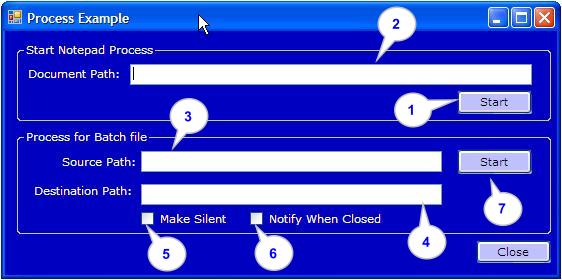
The sample application has two parts. The first part starts a Notepad process when the “Start” (marked as 1) button is clicked. You can enter the text document location in the TextBox (marked as 2) before clicking the start button. When a text document is specified, the notepad process opens that document. In the second part, a batch file is began as the process by clicking the start button (marked as 7). The batch file actually does a xcopy command that requires source and destination locations. The data can be supplied using two text boxes (marked as 3 and 4). When a batch file execution continues you can see a console window in the background. The checkbox “Make Silent” (marked as 5) suppresses the background command window. The checkbox “Notify when closed” (marked as 6) when checked, allows the application receiving the notification when the process finished its operation.
Starting the Notepad Process
A program is an executable stored in a physical disc. Say for example Notepad.exe stored in the Windows System32 folder is called the Notepad program. When the program in secondary storage (Disc) loads into primary storage for the execution of instructions set in it, we call it a process. Windows has the capability of spawning multiple processes at a time and we call Windows a multi-tasking operating system. When all processes running in the background they compete for the shared resources. OK, this article we will see how to spawn a process from a C# application.
About the Example
The following screen shot shows the application example:
The sample application has two parts. The first part starts a Notepad process when the “Start” (marked as 1) button is clicked. You can enter the text document location in the TextBox (marked as 2) before clicking the start button. When a text document is specified, the notepad process opens that document. In the second part, a batch file is began as the process by clicking the start button (marked as 7). The batch file actually does a xcopy command that requires source and destination locations. The data can be supplied using two text boxes (marked as 3 and 4). When a batch file execution continues you can see a console window in the background. The checkbox “Make Silent” (marked as 5) suppresses the background command window. The checkbox “Notify when closed” (marked as 6) when checked, allows the application receiving the notification when the process finished its operation.
Starting the Notepad Process
To create a process, we need to use the Process class that can be found in the System.Diagnostics namespace. The following is the using statement for it.
- //Sample 01: Use Diagnostics namespace
- using System.Diagnostics;
- //Sample 03: Start Notepad process.
- private void btnStartNotepad_Click(object sender, EventArgs e)
- {
- if (txtDocPath.Text == String.Empty) Process.Start("Notepad.exe");
- else Process.Start("Notepad.exe", txtDocPath.Text);
- }
This demo application starts the “copy.bat” batch file as a Process. The content of the copy.bat is shown below.
The batch file actually copies one or more files or directories from the specified source to the specified destination. The source and destination locations can be passed as parameters to the batch file and these parameters will be substituted from the %1 and %2 in the batch file. Our C# example application passes these parameters as arguments to the Process and we will see that in the next session.
Running a batch file as a process
The code explanation below describes how a batch file can be run as a process.
First, the process is declared as a private data member of the class. The code is given below.
- //Sample 02: Process for the batch
- private Process BatchProc = null;
- //4.1: Return when the strings are empty. However, path validation not done
- if (txtSrcPath.Text == string.Empty || txtDestPath.Text == string.Empty)
- return;
- //4.2: Populate Process Information
- ProcessStartInfo ProcInfo = new ProcessStartInfo();
- ProcInfo.FileName = "Copy.bat";
- ProcInfo.Arguments = "\"" + txtSrcPath.Text + "\" " + "\"" + txtDestPath.Text + "\"";
The Yellow highlighted double quotes are required since some paths may contain spaces. In the preceding picture D:\Temp does not have any space but the path in argument 1 has the space. Since the inputs are taken from the UI, it is advisable to use the escape sequence when forming the Argument string. Parameter1 and parameter2 are separated by a blank space.
Once the ProcessStartInfo structure is ready, we can pass that structure to the Start method call of the Process class. This is shown below.
- //4.4: Start the Process and Hook-up process Exit Event
- BatchProc = Process.Start(ProcInfo);
Process – Suppress Background
Sometimes we need to start a process in silent mode. That means, the application responsible for starting the process should not display any background window and the process task should go silent. Setting the hidden WindowStyle to the ProcessStartInfo class can do this. The code for starting the process silent is given below.
- //4.3: Start the process in silent Mode3
- if (chkBoxSilent.Checked == true)
- {
- ProcInfo.WindowStyle = ProcessWindowStyle.Hidden;
- }
In some cases we need to get a notification from the process stating the task completed or process exited. In our case the process exits when the task of copying the file from source to destination is completed. The application starting the process can handle the “Exited“ event of the process to get the notification from the process. The code is given below.
- if (chkWhenClosed.Checked == true)
- {
- BatchProc.EnableRaisingEvents = true;
- BatchProc.Exited += new System.EventHandler(Batch_Done);
- }
- //Sample 05: Handle Batch completed event
- private void Batch_Done(object sender, EventArgs e)
- {
- MessageBox.Show("Copy Completed!");
- BatchProc.Exited -= new System.EventHandler(Batch_Done);
- }
No comments:
Post a Comment